03 Teach : Team Activity
Parsing JSON
Objectives
Become familiar with JSON.
Understand how to deserialize JSON into objects and collections.
Assignment
Part I: Understanding JSON
JSON is a data storage format originally developed for web applications. It has become one of the most popular data exchange formats because of its compact size and readability. (Another popular data format is XML. If you're interested, you can read more about the pros and cons of the two here.)
Here is what an array looks like in JSON:
// Array of numbers
[ 1, 2, 3, 4]
// Array of strings
[ "mon", "tue", "wed", "thu", "fri", "sat", "sun"]
// Array of booleans:
[ true, false, true, true]
JSON stands for "JavaScript Object Notation". Even though we can use it to store simple arrays, it's designed to allow complex objects to be stored in key-value pairs.
The keys are strings that correspond to the property names, and the values are the values of the property for a particular object:
// Player object
Player p = new Player();
p.setName("Steve");
p.setHealth(20);
p.setMana(50);
p.setCanFly(false);
// JSON Representation
{ "name": "Steve", "health": 20, "mana": 50, "canFly": false}
We can take an object, serialize into JSON, and then share that data, (either in a file or by sending it over the network. Then we can deserialize it back into an object.
Part II: Create a Project with the GSON Library
Unfortunately, Java doesn't have built-in support for JSON, so to parse it we have to use a 3rd party library. There are several available, but we'll be using Google's GSON library (GSON just stands for "Google JSON", it isn't a different data format).
We'll be using a pre-compiled jar version of the library. A jar file is like a zip file containing a bunch of compiled java classes. It's how java programs and libraries are typically shared.
Maven is a popular place to store 3rd party libraries. IntelliJ can load libraries directly from Maven pretty easily.
-
To begin, create a new command line project in IntelliJ.
-
After the project loads, add the GSON library from Maven. If you're not sure how to do this, you might need to google: intellij add library from maven and look at the official docs on the JetBrains website.
You should use the most recent version of GSON, at this time, that is: com.google.code.gson:gson:2.8.5.
-
Test that the library has been installed correctly by trying to use the
Gson
class inmain()
and making sure it compiles without errors:package teach03; import com.google.gson.Gson; public class Main { public static void main(String[] args) { Gson g = new Gson(); } }
If you got an error, about not being able to find the Gson library, you'll need to go back to step 2 and try to add it again.
Part III: Use the GSON library.
With the GSON library installed, it's now time to try serializing and deserializing some things.
Note that this part will be a bit difficult, as I'm leaving many of the details up to you to figure out.
You may want to refer to the GSON tutorials here as you go through the rest of this assignment.
-
Create a class definition for a class called
Player
that has properties for name, health, mana, and gold.You might also want to add some kind of display function you can use to easily show those values.
-
Create a class called
Game
that will take an instance of thePlayer
class in its constructor and store it in a member variable. -
Create a function in the
Game
class calledsaveGame()
that will use the GSON library to serialize the object to a string, and save that string to a file. -
Add a static function to the
Game
class calledloadGame()
that takes a filename as a String and returns a newGame
generated from the data in that file.This function should load the file contents as a String, then use the GSON library to deserialize it into a new Player class, create a new
Game
instance with that player, then return the new game instance. -
In
main()
, create an instance of thePlayer
class, and populate its values. Then, create a new instance of theGame
class, passing the player you created to its constructor. Then call thesaveGame()
method. Verify the save file contains the correct JSON data. -
Use the
loadGame()
function of theGame
class you created in order to create a secondGame
object, passing in the filename you used in the previous step. Verify that thePlayer
object contained in the new game has been loaded correctly. Its values should match those of the original.
Once you've made it this far, move on the stretch challenge.
🌟Stretch Challenge🌟
The following is a UML diagram of how the class relationships might look after you complete the Stretch Challenge.
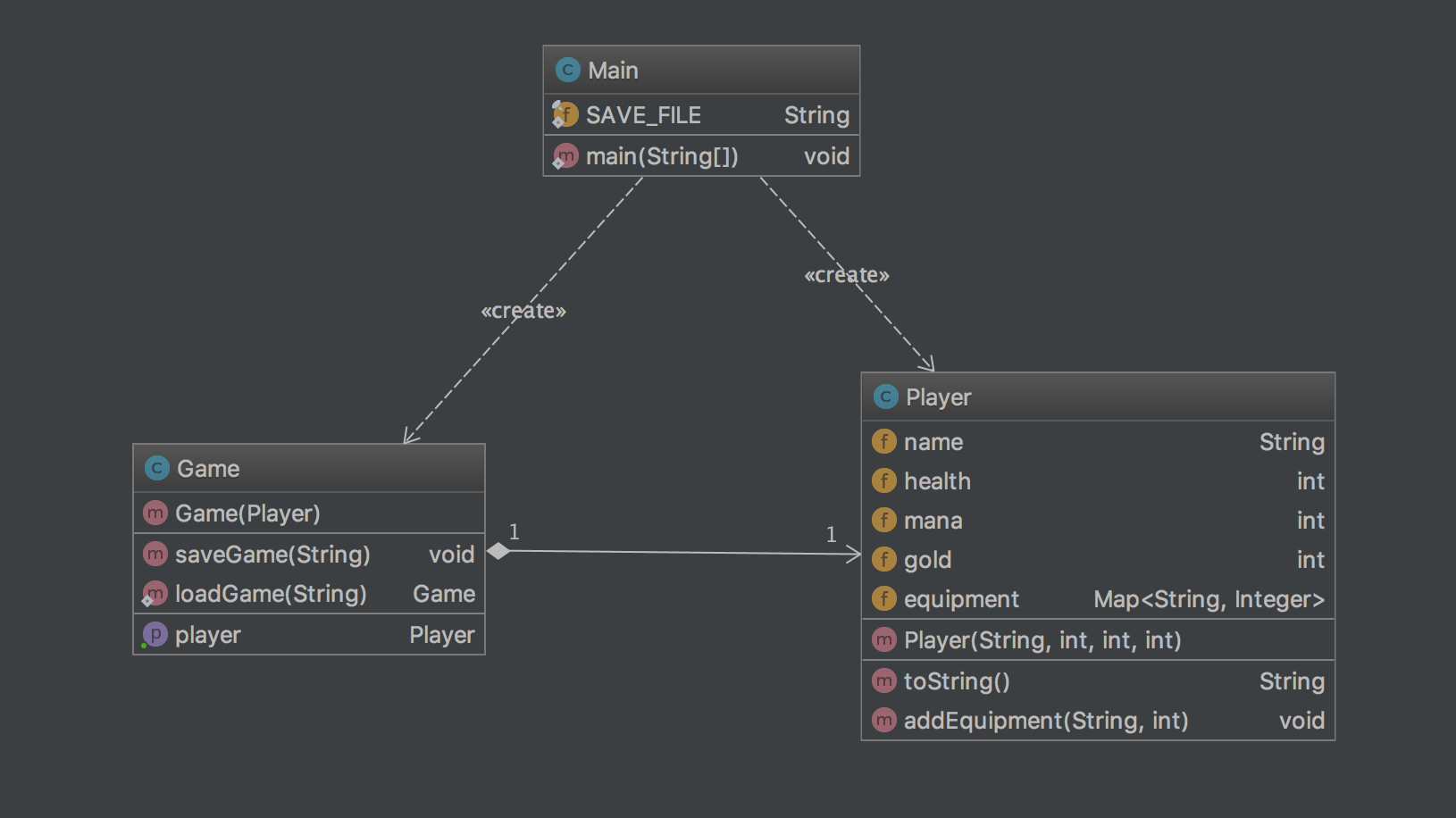
Let's allow our player to have some equipment.
-
Add a
Map
calledequipment
to thePlayer
class that will map equipment names as Strings to equipment costs as Integers. -
Modify
main()
so that it will add some equipment to thePlayer
instance before passing it to theGame
constructor. Make sure you're following good rules of encapsulation here and not acessing theMap
directly frommain()
. -
Verify that you can still serialize and deserialize the complete
Player
object (including euqipment), and if not, modify your code as needed to make it work.
Congratulations! You now know how to serialize and deserialize with JSON.
Make sure everyone on your team understands and receives a copy of this code.
Compare your answer to the teacher's solution, then complete the accompanying quiz for this assignment on I-Learn.